Why you should use Typescript
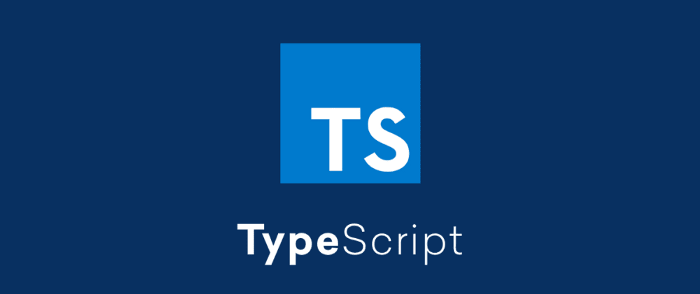
I have a confession to make; I was dead set against using Typescript because it came from Microsoft. I remember when I first started hearing about the project, I immediately dismissed it. Yes, Javascript has some (many) rough edges. But the Lord saw fit to give John Resig the idea for JQuery and that sort of smoothed over things. I mean, there was a time on the web, when literally every website had the dollar sign global variable in the code.
So, how could Microsoft — a company that gave the world Internet Explorer 6 — do anything to help the web move forward?

But Typescript is different. I was and am being proved wrong by how useful this tool is. For a better breakdown of what I am talking about, let's quickly go over the issues (as many devs see it) that Javascript has.
The Problem with Javascript
Ok, so where to start?
The weird inheritance mechanism. Why prototypical and not classical hierarchy as seen in C++?
No data hiding. There is no such thing as private, protected, and public variables/methods in JS. There are no modules inherit in the language, so you can't even group functions by module. You have just to put a bunch of related stuff in the same file or same object and just make sure nothing else rewrites your variable name, since everything is global by default.
Yes, JS is a dynamic language and all languages in this family suffer from duck typing in some sort of way. But JS has that whole null and undefined types and it's honestly just a real mess.
The lack of modules makes it really hard to not end up in a mess of spaghetti. There are a bunch of projects that tried to solve this issue, but Typescript does the best and most elegant way.

What Typescript gets right
When you have a large codebase, you quickly realize how important organization is to a project. When you start adding more people looking at that project, you start to realize how nice it would be to have to code self-document. In this area, Typescript gets it right. You can just add the following and now you have a module ready to go.
# contents of mylib.js
exports function add(int x, int y) { return x + y; }
exports function sub(int x, int y) { return x - y; }
# contents of main.js
import { add, sub } from 'mylib'
add(1, 1);
sub(2, 3);
You can create real classes and will work as they do in 99% of the other major programming languages (no, Lisp doesn't count).
class A {
name: string
constructor(name){
this.name = name
}
public doSomething() {
return `{this.name} is doing something...`
}
}
class SubA extends A {
duration: Number
constructor(name, duration) {
super()
this.duration = duration;
}
public doSomething() {
return `{this.name} is going to be busy for {this.duration}`
}
}
You even get to use interfaces which opens up a lot of different design patterns that are clunky/not worth the effort to build out in regular old Javascript. If you don't know about interfaces and how they relate to code organization, do yourself a favor and read up on it. Interfaces are essentially contracts between pieces of code outlining either variables or methods that are going to be available to be used by the calling code.
interface Action {
name: string,
doSomething(int hitPoints)
}
class JumpUp implements Action {
name: string = "Jumping Up"
public doSomething(int hitPoints) {
return `{$this.name} will cost {$hitPoints}`
}
}
class GetDown implements Action {
name: string
constructor(name) {
this.name = name
}
public doSomething(int hitPoints) {
hitPoints = hitPoints * 2
return `{$this.name} will net you ${hitPoints}`
}
}
let thingToDo = new JumpUp()
let otherThingToDo = new GetDown()
function executeAction(Action thingToDo) {
return thingToDo.doSomething()
}
executeAction(thingToDo)
executeAction(otherThingToDo)
Hopefully, this gives you an idea of the power of interfaces. The calling code executeAction
didn't have to know whether it was a GetDown
class or a JumpUp
class that it was working on. All it needed to know is that whatever parameter is being sent to it, will have a method called doSomething
. That this the contract that I spoke of earlier. The interface is the common element that both classes have in common and that is the only part that the calling code needs to be aware of. This makes your codebase easier to reason and to explain to others.
And don't forget about the tooling
The other part is that there is a lot of different tooling that makes it easy to get started. Microsoft is not known for its developer experience in using any of their tools, but they did a good job with Typescript. Not only that but because Typescript is open source, the community of users have banded together to created a bounty of starter kits and commandline tools to faciliate development.